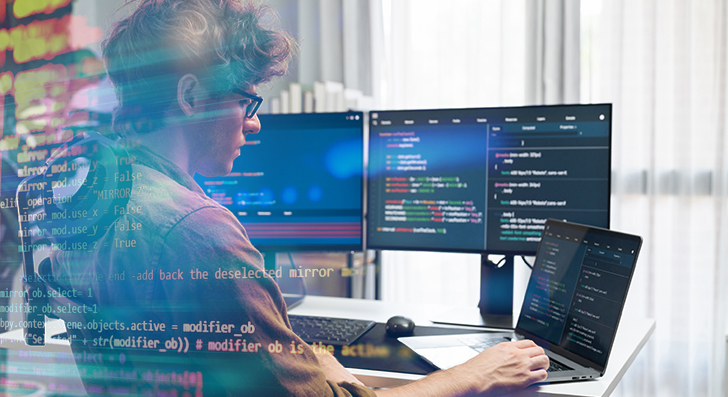
Scalability indicates your application can manage development—more buyers, additional info, and even more visitors—without breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on afterwards—it should be section of the approach from the beginning. Lots of programs are unsuccessful after they improve quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to think early about how your procedure will behave under pressure.
Start off by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Each module or support can scale By itself without impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle 1,000,000 end users or merely 100? Pick the right kind—relational or NoSQL—determined by how your facts will mature. Strategy for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Think about what would happen In case your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use design and style styles that support scaling, like concept queues or celebration-pushed units. These help your app handle more requests without obtaining overloaded.
Once you Make with scalability in your mind, you are not just preparing for success—you are lowering foreseeable future head aches. A nicely-planned procedure is simpler to keep up, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the appropriate databases can be a crucial A part of setting up scalable apps. Not all databases are developed exactly the same, and utilizing the Mistaken one can gradual you down or maybe lead to failures as your app grows.
Start out by comprehension your information. Can it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to deal with additional site visitors and details.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling substantial volumes of unstructured or semi-structured knowledge and can scale horizontally far more easily.
Also, take into account your read and compose designs. Have you been executing plenty of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Consider databases that could tackle higher compose throughput, or maybe party-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also intelligent to Consider in advance. You might not have to have Sophisticated scaling functions now, but selecting a database that supports them suggests you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your facts depending on your access patterns. And always keep track of database overall performance as you develop.
In brief, the correct databases is dependent upon your application’s construction, pace requirements, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save loads of problems later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay adds up. Improperly published code or unoptimized queries can slow down efficiency and overload your method. That’s why it’s important to Establish economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most advanced Resolution if a simple a person performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Every single query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing a lot of joins, Specifically throughout large tables.
In case you see the identical facts becoming requested time and again, use caching. Store the outcome quickly using resources like Redis or Memcached therefore you don’t really have to repeat costly operations.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more successful.
Make sure to test with big datasets. Code and queries that perform wonderful with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools aid maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out all click here of the work, the load balancer routes buyers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When end users request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it through the cache.
There are two prevalent varieties of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching decreases databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. With each other, they assist your application deal with far more buyers, remain rapidly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To construct scalable programs, you require tools that let your app increase quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to purchase hardware or guess potential capability. When targeted traffic boosts, you may increase extra resources with just some clicks or automatically using automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it needs to operate—code, libraries, options—into 1 unit. This can make it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your application works by using several containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it automatically.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy simply, and recover speedily when problems come about. If you would like your application to mature without having restrictions, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on building, not fixing.
Watch Everything
In case you don’t observe your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is carrying out, place difficulties early, and make improved decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just observe your servers—monitor your application too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This helps you fix challenges speedy, generally in advance of end users even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it brings about actual damage.
As your application grows, site visitors and information maximize. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you stay on top of things.
In short, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the ideal equipment, you could Construct applications that develop efficiently without breaking under pressure. Get started little, Consider big, and Construct clever.